前言
ArrayBlockingQueue
是采用数组实现的有界阻塞线程安全队列。如果向已满的队列继续塞入元素,将导致当前的线程阻塞。如果向空队列获取元素,那么将导致当前线程阻塞。
ArrayBlockingQueue
继承 AbstractQueue
类,实现 BlockingQueue
和 Serializable
接口,关于 AbstractQueue
和 BlockingQueue
的内容可以参考 抽象队列与阻塞队列解析
1 | public class ArrayBlockingQueue<E> extends AbstractQueue<E> |
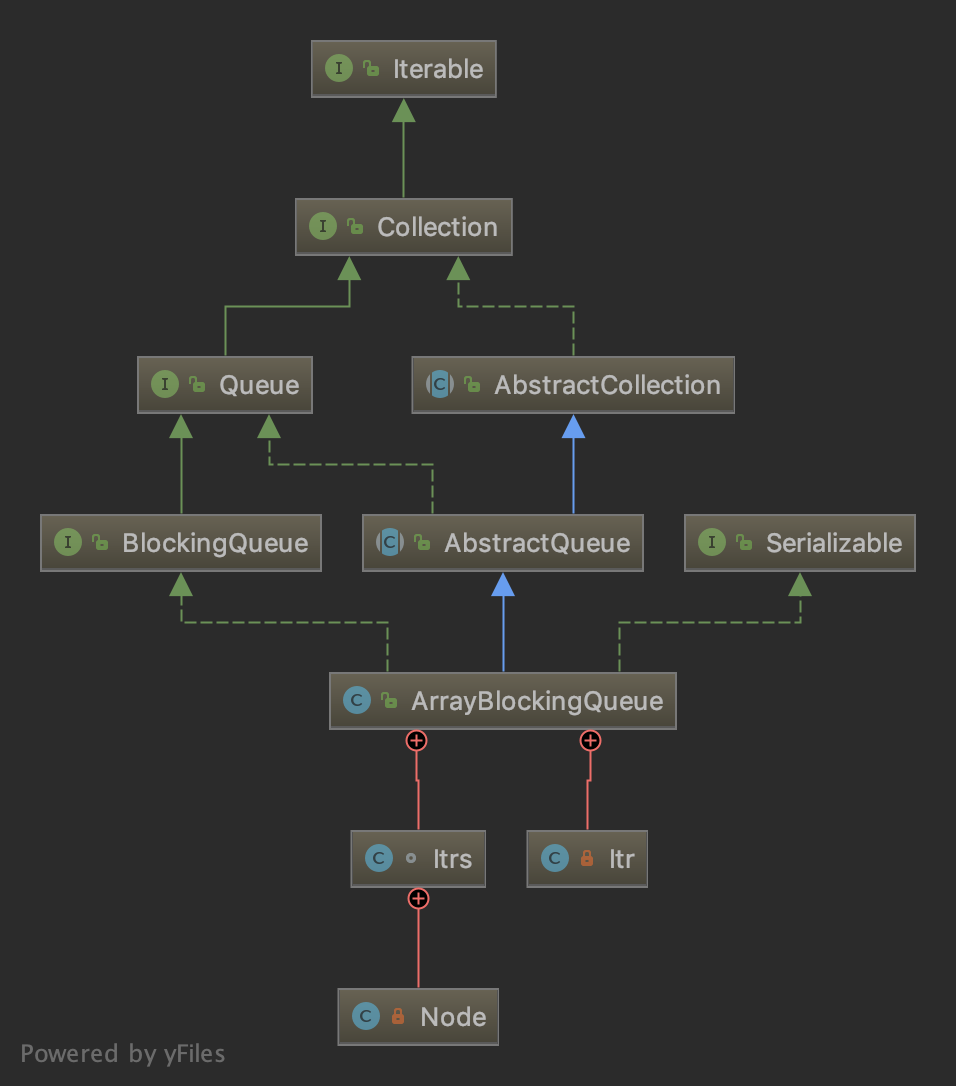
ArrayBlockingQueue
有两个内部类,分别为 Itrs
和 Itr
。其中 Itrs
内部类 Node
继承 WeakReference
类。Itr
实现了 Iterator
接口
使用示例
实现简单的生产者消费者模型:
1 | public class ArrayBlockingQueueTest { |
其中一次执行结果如下:
1 | Thread-4 provider : Food {name:Apple} |
类结构源码分析
类属性
可重入锁的内容可以参考 ReentrantLock解析
1 | /** 存放队列元素的数组 */ |
构造器
构造器有三种
1 | // 初始化阻塞队列容量,默认是采用不公平重入锁 |
内部类源码分析
Itrs内部类
1 | class Itrs { |
Itr 内部类
1 | private class Itr implements Iterator<E> { |
进入detach模式的关键有3种情况:
- cursor == putIndex,这时候 cursor = NONE
- 空队列
- cycle - preCycles > 1
常用操作源码分析
入队
1 | // 调用抽象队列的 add 方法 |
出队
1 | // 遍历并移除队列头部元素,不感知中断 |
转数组
1 | // 将队列转成 Object 数组 |
其他操作
1 | // 获取阻塞队列元素数量 |
特殊方法
将队列元素添加到集合
1 | // 将队列元素添加到集合 c,最大数量为 Integer.MAX_VALUE |
出队与入队
1 | // 入队 |
分割迭代器
1 | // jdk8新增,可用来并行遍历元素的一个迭代器 |
总结
ArrayBlockingQueue
采用数组实现的有界阻塞线程安全队列,是规定大小的 BlockingQueue
,其构造必须指定大小。其所含的对象是 FIFO 顺序排序的。